Edge Detector
This example performs edge detection on 3 different inputs: left, right and RGB camera. HW accelerated sobel filter 3x3 is used. Sobel filter parameters can be changed by keys 1 and 2.Demo
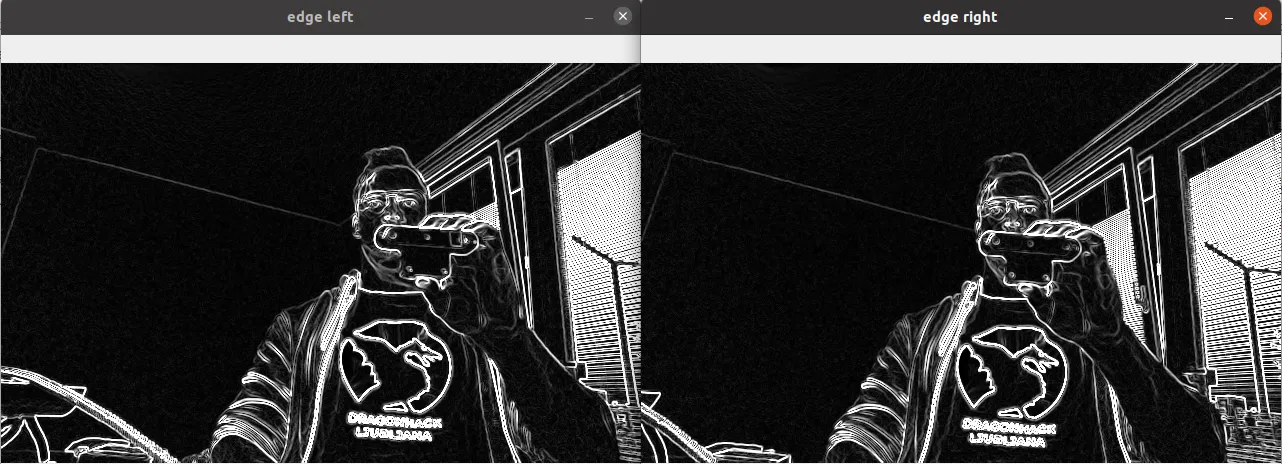
Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2
3import cv2
4import depthai as dai
5import numpy as np
6
7# Create pipeline
8pipeline = dai.Pipeline()
9
10# Define sources and outputs
11camRgb = pipeline.create(dai.node.ColorCamera)
12monoLeft = pipeline.create(dai.node.MonoCamera)
13monoRight = pipeline.create(dai.node.MonoCamera)
14
15edgeDetectorLeft = pipeline.create(dai.node.EdgeDetector)
16edgeDetectorRight = pipeline.create(dai.node.EdgeDetector)
17edgeDetectorRgb = pipeline.create(dai.node.EdgeDetector)
18
19xoutEdgeLeft = pipeline.create(dai.node.XLinkOut)
20xoutEdgeRight = pipeline.create(dai.node.XLinkOut)
21xoutEdgeRgb = pipeline.create(dai.node.XLinkOut)
22xinEdgeCfg = pipeline.create(dai.node.XLinkIn)
23
24edgeLeftStr = "edge left"
25edgeRightStr = "edge right"
26edgeRgbStr = "edge rgb"
27edgeCfgStr = "edge cfg"
28
29xoutEdgeLeft.setStreamName(edgeLeftStr)
30xoutEdgeRight.setStreamName(edgeRightStr)
31xoutEdgeRgb.setStreamName(edgeRgbStr)
32xinEdgeCfg.setStreamName(edgeCfgStr)
33
34# Properties
35camRgb.setBoardSocket(dai.CameraBoardSocket.CAM_A)
36camRgb.setResolution(dai.ColorCameraProperties.SensorResolution.THE_1080_P)
37
38monoLeft.setResolution(dai.MonoCameraProperties.SensorResolution.THE_400_P)
39monoLeft.setCamera("left")
40monoRight.setResolution(dai.MonoCameraProperties.SensorResolution.THE_400_P)
41monoRight.setCamera("right")
42
43edgeDetectorRgb.setMaxOutputFrameSize(camRgb.getVideoWidth() * camRgb.getVideoHeight())
44
45# Linking
46monoLeft.out.link(edgeDetectorLeft.inputImage)
47monoRight.out.link(edgeDetectorRight.inputImage)
48camRgb.video.link(edgeDetectorRgb.inputImage)
49
50edgeDetectorLeft.outputImage.link(xoutEdgeLeft.input)
51edgeDetectorRight.outputImage.link(xoutEdgeRight.input)
52edgeDetectorRgb.outputImage.link(xoutEdgeRgb.input)
53
54xinEdgeCfg.out.link(edgeDetectorLeft.inputConfig)
55xinEdgeCfg.out.link(edgeDetectorRight.inputConfig)
56xinEdgeCfg.out.link(edgeDetectorRgb.inputConfig)
57
58# Connect to device and start pipeline
59with dai.Device(pipeline) as device:
60
61 # Output/input queues
62 edgeLeftQueue = device.getOutputQueue(edgeLeftStr, 8, False)
63 edgeRightQueue = device.getOutputQueue(edgeRightStr, 8, False)
64 edgeRgbQueue = device.getOutputQueue(edgeRgbStr, 8, False)
65 edgeCfgQueue = device.getInputQueue(edgeCfgStr)
66
67 print("Switch between sobel filter kernels using keys '1' and '2'")
68
69 while(True):
70 edgeLeft = edgeLeftQueue.get()
71 edgeRight = edgeRightQueue.get()
72 edgeRgb = edgeRgbQueue.get()
73
74 edgeLeftFrame = edgeLeft.getFrame()
75 edgeRightFrame = edgeRight.getFrame()
76 edgeRgbFrame = edgeRgb.getFrame()
77
78 # Show the frame
79 cv2.imshow(edgeLeftStr, edgeLeftFrame)
80 cv2.imshow(edgeRightStr, edgeRightFrame)
81 cv2.imshow(edgeRgbStr, edgeRgbFrame)
82
83 key = cv2.waitKey(1)
84 if key == ord('q'):
85 break
86
87 if key == ord('1'):
88 print("Switching sobel filter kernel.")
89 cfg = dai.EdgeDetectorConfig()
90 sobelHorizontalKernel = [[1, 0, -1], [2, 0, -2], [1, 0, -1]]
91 sobelVerticalKernel = [[1, 2, 1], [0, 0, 0], [-1, -2, -1]]
92 cfg.setSobelFilterKernels(sobelHorizontalKernel, sobelVerticalKernel)
93 edgeCfgQueue.send(cfg)
94
95 if key == ord('2'):
96 print("Switching sobel filter kernel.")
97 cfg = dai.EdgeDetectorConfig()
98 sobelHorizontalKernel = [[3, 0, -3], [10, 0, -10], [3, 0, -3]]
99 sobelVerticalKernel = [[3, 10, 3], [0, 0, 0], [-3, -10, -3]]
100 cfg.setSobelFilterKernels(sobelHorizontalKernel, sobelVerticalKernel)
101 edgeCfgQueue.send(cfg)
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.