SpatialLocationCalculator
SpatialLocationCalculator node calculates the spatial coordinates of the ROI (region-of-interest) based on thedepth
map from the inputDepth
. It will average the depth values in the ROI and remove the ones out of range.You can also calculate spatial coordinates on host side, demo here. The demo also has the same logic that's performed on the device (calc.py
file).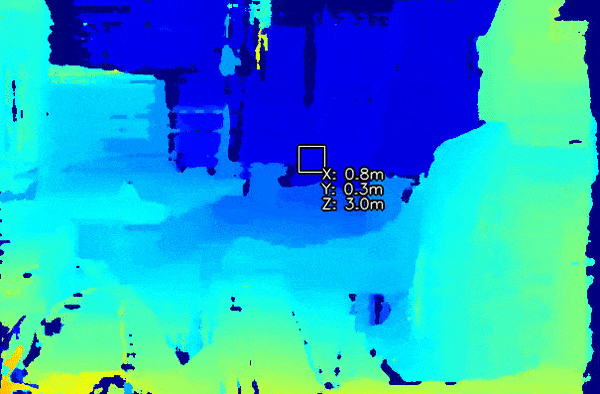
How to place it
Python
C++
Python
Python
1pipeline = dai.Pipeline()
2spatialCalc = pipeline.create(dai.node.SpatialLocationCalculator)
Inputs and Outputs
Usage
Python
C++
Python
Python
1pipeline = dai.Pipeline()
2spatialCalc = pipeline.create(dai.node.SpatialLocationCalculator)
3spatialCalc.setWaitForConfigInput(False)
4
5# Set initial config
6config = dai.SpatialLocationCalculatorConfigData()
7config.depthThresholds.lowerThreshold = 100
8config.depthThresholds.upperThreshold = 10000
9
10topLeft = dai.Point2f(0.4, 0.4)
11bottomRight = dai.Point2f(0.6, 0.6)
12config.roi = dai.Rect(topLeft, bottomRight)
13
14spatial_calc.initialConfig.addROI(config)
15
16# You can later send configs from the host (XLinkIn) / Script node to the InputConfig
Examples of functionality
Spatial coordinate system
OAK camera uses left-handed (Cartesian) coordinate system for all spatial coordinates.Reference
class
depthai.node.SpatialLocationCalculator(depthai.Node)
method
getWaitForConfigInput(self) -> bool: bool
See also: setWaitForConfigInput Returns: True if wait for inputConfig message, false otherwise
method
setWaitForConfigInput(self, wait: bool)
Specify whether or not wait until configuration message arrives to inputConfig Input. Parameter ``wait``: True to wait for configuration message, false otherwise.
property
initialConfig
Initial config to use when calculating spatial location data.
property
inputConfig
Input SpatialLocationCalculatorConfig message with ability to modify parameters in runtime. Default queue is non-blocking with size 4.
property
inputDepth
Input message with depth data used to retrieve spatial information about detected object. Default queue is non-blocking with size 4.
property
out
Outputs SpatialLocationCalculatorData message that carries spatial location results.
property
passthroughDepth
Passthrough message on which the calculation was performed. Suitable for when input queue is set to non-blocking behavior.
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.