ToF
ToF node is used for converting the raw data from the ToF sensor into a depth map. Currently, these 2 products contain a ToF sensor:- OAK-D SR PoE - integrated 33D ToF sensor, together with a stereo camera pair
- OAK-FFC ToF 33D - standalone FFC module with a 33D ToF sensor
depth
output can be used instead of StereoDepth's - so you can link ToF.depth
to MobileNetSpatialDetectionNetwork/YoloSpatialDetectionNetwork or SpatialLocationCalculator directly.ToF's depth accuracy is, in general, more accurate compared to Stereo depth. You can see ToF depth accuracy here.ToF pointcloud demo video, showcasing the depth accuracy:How to place it
Python
C++
Python
Python
1pipeline = dai.Pipeline()
2tof = pipeline.create(dai.node.ToF)
Inputs and Outputs
ToF Settings
In ToF depth example we allow users to quickly configure ToF settings.Here are the most important settings:- Optical Correction: It's a process that corrects the optical effect. When enabled, the ToF returns depth map (represented by Green Line on graph below) instead of distance, so it matches StereoDepth depth reporting. It does rectification and distance to depth conversion (Z-map).
- Undistortion:
depth
andamplitude
frames are undistorted by default. - Phase Unwrapping - Process that corrects the phase wrapping effect of the ToF sensor. You can set it to [0..5 are optimized]. The higher the number, the longer the ToF range, but it also increases the noise. Approximate max distance (for exact value, see Max distance below):
0
- Disabled, up to ~1.87 meters (utilizing 80MHz modulation frequency)1
- Up to ~3 meters2
- Up to ~4.5 meters3
- Up to ~6 meters4
- Up to ~7.5 meters
- Burst mode: When enabled, ToF node won't reuse frames, as shown on the graph below. It's related to post-processing of the ToF frames, not the actual sensor/projector. It's disabled by default.
- Phase shuffle Temporal filter: Averages shuffled and non-shuffled frames of the same modulation frequency to reduce noise. It's enabled by default. You can disable it to reduce ToF motion blur and system load.
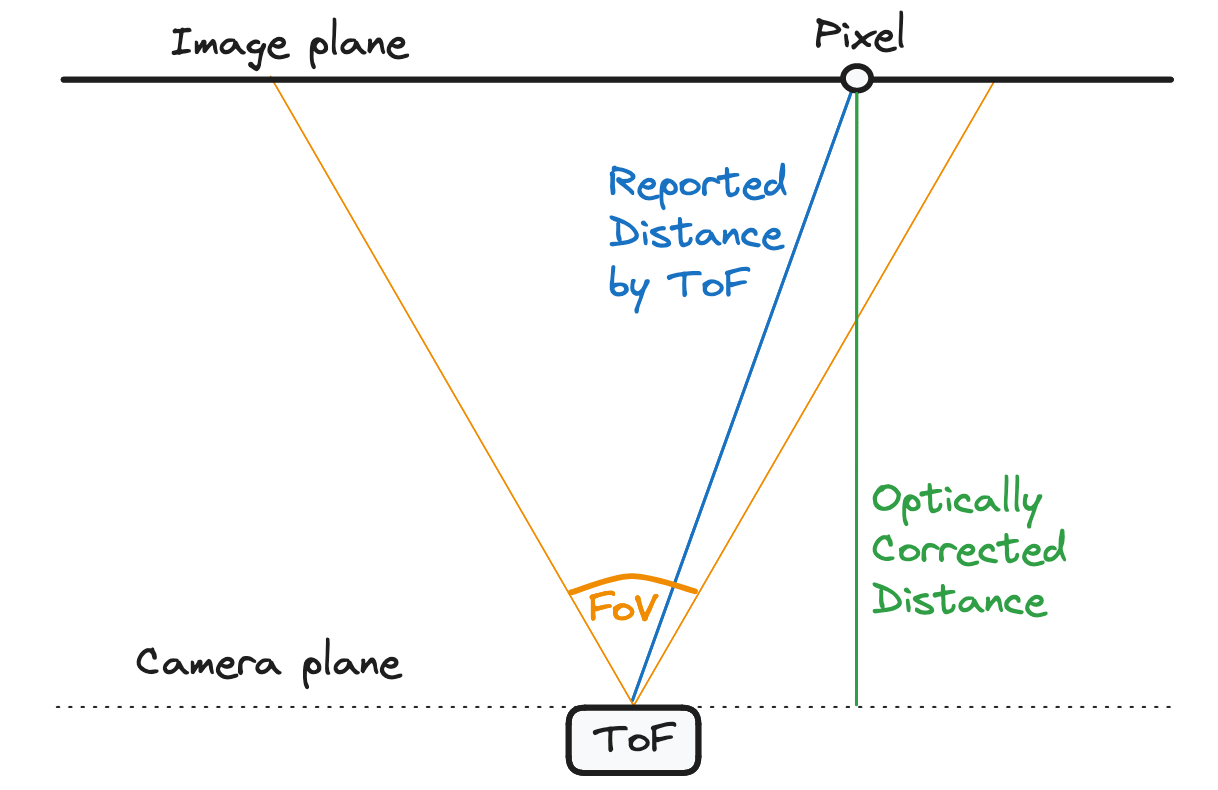
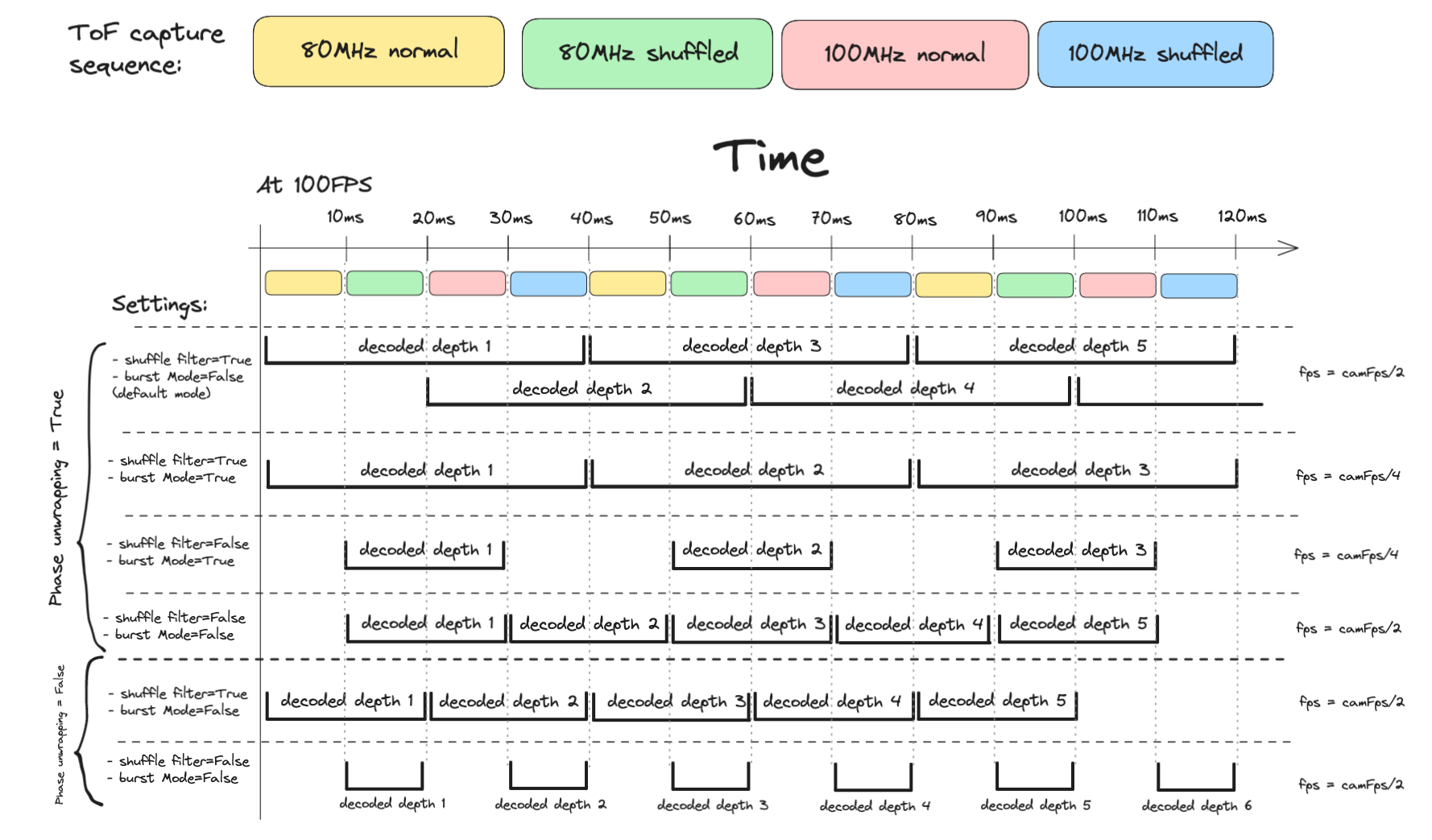
Phase unwrapping
If the time it takes for the light to travel from ToF sensor and back exceeds the period of the emitted wave (1.5m or 1.87m), the resulting measurement will "wrap" back to a lower value. This is called phase wrapping. It's similar to how a clock resets after 12 hours. Phase unwrapping is possible as our ToF has two different modulation frequencies (80Mhz and 100MHz).Phase unwrapping aims to correct this by allowing the sensor to interpret longer distances without confusion. It uses algorithms to keep track of how many cycles (round trips of the wave) have occurred, thus correcting the "wrapped" phases. The downside is that the more cycles the sensor has to keep track of, the more noise it introduces into the measurement.ToF motion blur
To reduce motion blur, we recommend these settings:- Increase camera FPS. It goes up to 160 FPS, which causes frame capture to be the fastest (6.25ms between frames). This will reduce motion blur as ToF combines multiple frames to get the depth. Note that 160FPS will increase system load significantly (see Debugging DepthAI pipeline). Note also that higher FPS -> lower exposure times, which can increase noise.
- Disable phase shuffle temporal filter. This will introduce more noise.
- Disable phase unwrapping. This will reduce max distance to 1.87 meters (utilizing 80MHz modulation frequency), so about 1 cubic meter of space will be visible (very limited use-cases).
- Enable burst mode. This is irrelevant if shuffle filter and phase unwrapping are disabled (see diagram above). When enabled, ToF node won't reuse frames (lower FPS).
Max distance
Maximum ToF distance depends on the modulation frequency and the phase unwrapping level. If phase unwrapping is enabled, max distance is the shorter of both modulation frequencies (so max distance at 100MHz). Here's the formula:Math
1c = 299792458.0 # speed of light in m/s
2
3MAX_80MHZ_M = c / (80000000 * 2) = 1.873 m
4MAX_100MHZ_M = c / (100000000 * 2) = 1.498 m
5
6MAX_DIST_80MHZ_M = (phaseUnwrappingLevel + 1) * 1.873 + (phaseUnwrapErrorThreshold / 2)
7MAX_DIST_100MHZ_M = (phaseUnwrappingLevel + 1) * 1.498 + (phaseUnwrapErrorThreshold / 2)
8
9MAX_DIST_PHASE_UNWRAPPING_M = MAX_DIST_100MHZ_M
ToF FPS
Sensor/emitter can go up to 160 FPS, which will translte to depth output at either 40 FPS (burst mode enabled) or 80 FPS (burst mode disabled). This is due to different modulation frequencies (80MHz and 100MHz) and the need to combine shuffled/non-shuffled frames to reduce noise.Usage
Python
C++
Python
Python
1pipeline = dai.Pipeline()
2
3tof_cam = pipeline.create(dai.node.Camera)
4tof_cam.setFps(30)
5# We assume the ToF camera sensor is on port CAM_A
6tof_cam.setBoardSocket(dai.CameraBoardSocket.CAM_A)
7
8tof = pipeline.create(dai.node.ToF)
9
10# Higher number => faster processing. 1 shave core can do 30FPS.
11tof.setNumShaves(1)
12
13# Median filter, kernel size 5x5
14tof.initialConfig.setMedianFilter(dai.MedianFilter.KERNEL_5x5)
15
16tofConfig = tof.initialConfig.get()
17# Temporal filter averages shuffle/non-shuffle frequencies
18tofConfig.enablePhaseShuffleTemporalFilter = True
19# Phase unwrapping, for longer range.
20tofConfig.phaseUnwrappingLevel = 4 # Up to 7.5 meters
21tofConfig.phaseUnwrapErrorThreshold = 300
22tof.initialConfig.set(tofConfig)
23
24# ToF node converts raw sensor frames into depth
25tof_cam.raw.link(tof.input)
26
27# Send ToF depth output to the host, or perhaps to SLC / Spatial Detection Network
28tof.depth.link(xout.input)
Examples of functionality
- ToF depth - Example of getting ToF depth
- RGB-ToF Alignment - Align tof depth to color stream
Reference
class
depthai.node.ToF(depthai.Node)
method
setNumFramesPool(self, arg0: int) -> ToF: ToF
Specify number of frames in output pool Parameter ``numFramesPool``: Number of frames in output pool
method
setNumShaves(self, arg0: int) -> ToF: ToF
Specify number of shaves reserved for ToF decoding.
property
amplitude
Outputs ImgFrame message that carries amplitude image.
property
depth
Outputs ImgFrame message that carries decoded depth image.
property
initialConfig
Initial config to use for depth calculation.
property
input
Input raw ToF data. Default queue is blocking with size 8.
property
inputConfig
Input ToF message with ability to modify parameters in runtime. Default queue is non-blocking with size 4.
property
intensity
Outputs ImgFrame message that carries intensity image.
property
phase
Outputs ImgFrame message that carries phase image, useful for debugging. float32 type.
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.