ColorCamera
ColorCamera node is a source of ImgFrame. You can control in at runtime with theInputControl
and InputConfig
.How to place it
Python
C++
Python
Python
1pipeline = dai.Pipeline()
2cam = pipeline.create(dai.node.ColorCamera)
Inputs and Outputs
Detailed node connections
inputConfig
- ImageManipConfiginputControl
- CameraControlraw
- ImgFrame - RAW10 bayer data. Demo code for unpacking hereisp
- ImgFrame - YUV420 planar (same as YU12/IYUV/I420)still
- ImgFrame - NV12, suitable for bigger size frames. The image gets created when a capture event is sent to the ColorCamera, so it's like taking a photopreview
- ImgFrame - RGB (or BGR planar/interleaved if configured), mostly suited for small size previews and to feed the image into NeuralNetworkvideo
- ImgFrame - NV12, suitable for bigger size frames
video
/preview
/still
frames.still
(when a capture is triggered) and isp
work at the max camera resolution, while video
and preview
are limited to max 4K (3840 x 2160) resolution, which is cropped from isp
. For IMX378 (12MP), the post-processing works like this: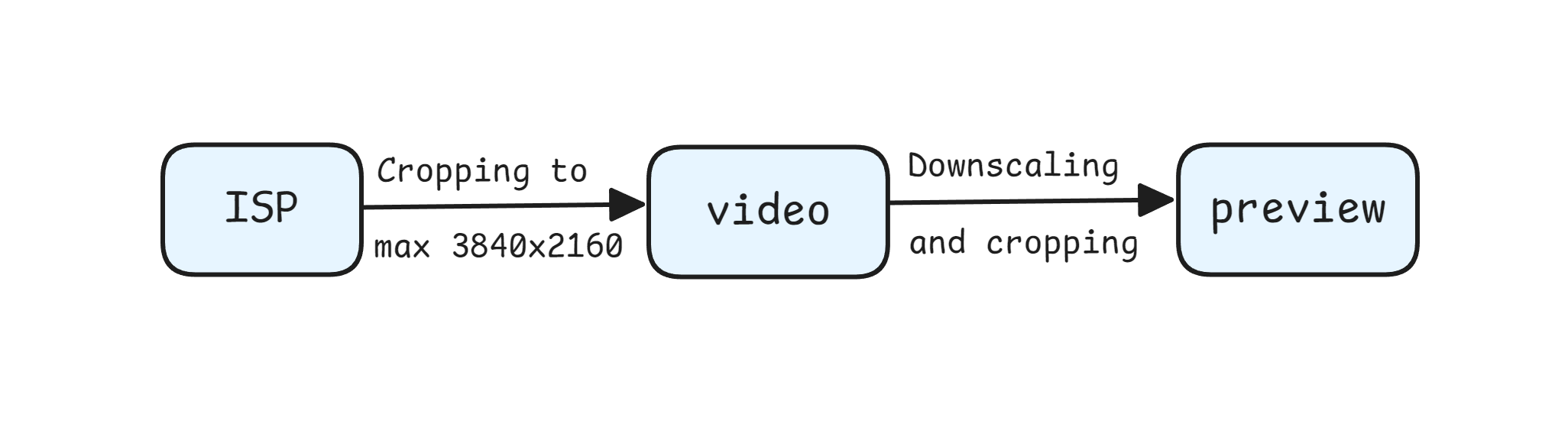
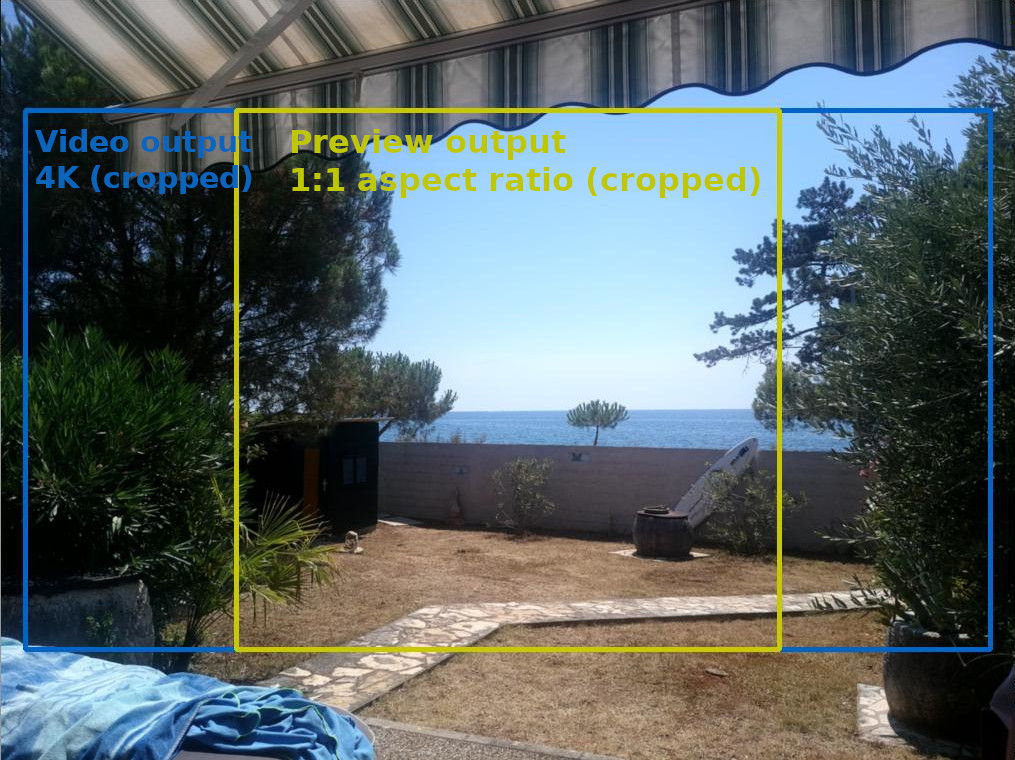
isp
output from the ColorCamera (12MP resolution from IMX378). If you aren't downscaling ISP, the video
output is cropped to 4k (max 3840x2160 due to the limitation of the video
output) as represented by the blue rectangle. The Yellow rectangle represents a cropped preview
output when the preview size is set to a 1:1 aspect ratio (eg. when using a 300x300 preview size for the MobileNet-SSD NN model) because the preview
output is derived from the video
output.Usage
Python
C++
Python
Python
1pipeline = dai.Pipeline()
2cam = pipeline.create(dai.node.ColorCamera)
3cam.setPreviewSize(300, 300)
4cam.setBoardSocket(dai.CameraBoardSocket.CAM_A)
5cam.setResolution(dai.ColorCameraProperties.SensorResolution.THE_1080_P)
6cam.setInterleaved(False)
7cam.setColorOrder(dai.ColorCameraProperties.ColorOrder.RGB)
3A Algorithms
The 3A - Auto-Exposure (AE), Auto-White Balance (AWB), and Auto-Focus (AF) - algorithms are used to optimize image quality and run directly on RVC. By default, these settings are in AUTO mode, with limits (e.g., min/max exposure) specific to each sensor (see supported sensors for details).You can manually control these settings either by following the steps in RGB camera control example or by using the cam_test.py script.- Stereo Cameras: Sensors share the same I2C bus, ensuring synchronized 3A settings automatically (AWB, AE).
- Independent Sensors: On setups like OAK FFC or OAK-D-LR, where each sensor has its own I2C, the
3a-follow
feature can be used to synchronize 3A settings from one sensor to others.
Python
1cam['cam_b'].initialControl.setMisc("3a-follow", dai.CameraBoardSocket.CAM_A)
2cam['cam_c'].initialControl.setMisc("3a-follow", dai.CameraBoardSocket.CAM_A)
3a-follow
feature copies the 3A settings (exposure, ISO, and white balance) from a primary camera (e.g., CAM_A) to other cameras in the setup (e.g., CAM_B and CAM_C).Limitations
Here are known camera limitations for the RVC2:- ISP can process about 600 MP/s, and about 500 MP/s when the pipeline is also running NNs and video encoder in parallel
- 3A algorithms can process about 200..250 FPS overall (for all camera streams). This is a current limitation of our implementation, and we have plans for a workaround to run 3A algorithms on every Xth frame, no ETA yet
- ISP Scaling numerator value can be 1..16 and denominator value 1..32 for both vertical and horizontal scaling. So you can downscale eg. 12MP (4056x3040) only to resolutions calculated here
Examples of functionality
Reference
class
depthai.node.ColorCamera(depthai.Node)
method
getBoardSocket(self) -> depthai.CameraBoardSocket: depthai.CameraBoardSocket
Retrieves which board socket to use Returns: Board socket to use
method
method
getCamera(self) -> str: str
Retrieves which camera to use by name Returns: Name of the camera to use
method
getColorOrder(self) -> depthai.ColorCameraProperties.ColorOrder: depthai.ColorCameraProperties.ColorOrder
Get color order of preview output frames. RGB or BGR
method
getFp16(self) -> bool: bool
Get fp16 (0..255) data of preview output frames
method
getFps(self) -> float: float
Get rate at which camera should produce frames Returns: Rate in frames per second
method
method
getImageOrientation(self) -> depthai.CameraImageOrientation: depthai.CameraImageOrientation
Get camera image orientation
method
getInterleaved(self) -> bool: bool
Get planar or interleaved data of preview output frames
method
getIspHeight(self) -> int: int
Get 'isp' output height
method
getIspNumFramesPool(self) -> int: int
Get number of frames in isp pool
method
getIspSize(self) -> tuple[int, int]: tuple[int, int]
Get 'isp' output resolution as size, after scaling
method
getIspWidth(self) -> int: int
Get 'isp' output width
method
getPreviewHeight(self) -> int: int
Get preview height
method
getPreviewKeepAspectRatio(self) -> bool: bool
See also: setPreviewKeepAspectRatio Returns: Preview keep aspect ratio option
method
getPreviewNumFramesPool(self) -> int: int
Get number of frames in preview pool
method
getPreviewSize(self) -> tuple[int, int]: tuple[int, int]
Get preview size as tuple
method
getPreviewWidth(self) -> int: int
Get preview width
method
getRawNumFramesPool(self) -> int: int
Get number of frames in raw pool
method
getResolution(self) -> depthai.ColorCameraProperties.SensorResolution: depthai.ColorCameraProperties.SensorResolution
Get sensor resolution
method
getResolutionHeight(self) -> int: int
Get sensor resolution height
method
getResolutionSize(self) -> tuple[int, int]: tuple[int, int]
Get sensor resolution as size
method
getResolutionWidth(self) -> int: int
Get sensor resolution width
method
getSensorCrop(self) -> tuple[float, float]: tuple[float, float]
Returns: Sensor top left crop coordinates
method
getSensorCropX(self) -> float: float
Get sensor top left x crop coordinate
method
getSensorCropY(self) -> float: float
Get sensor top left y crop coordinate
method
getStillHeight(self) -> int: int
Get still height
method
getStillNumFramesPool(self) -> int: int
Get number of frames in still pool
method
getStillSize(self) -> tuple[int, int]: tuple[int, int]
Get still size as tuple
method
getStillWidth(self) -> int: int
Get still width
method
getVideoHeight(self) -> int: int
Get video height
method
getVideoNumFramesPool(self) -> int: int
Get number of frames in video pool
method
getVideoSize(self) -> tuple[int, int]: tuple[int, int]
Get video size as tuple
method
getVideoWidth(self) -> int: int
Get video width
method
getWaitForConfigInput(self) -> bool: bool
See also: setWaitForConfigInput Returns: True if wait for inputConfig message, false otherwise
method
sensorCenterCrop(self)
Specify sensor center crop. Resolution size / video size
method
setBoardSocket(self, boardSocket: depthai.CameraBoardSocket)
Specify which board socket to use Parameter ``boardSocket``: Board socket to use
method
method
setCamera(self, name: str)
Specify which camera to use by name Parameter ``name``: Name of the camera to use
method
setColorOrder(self, colorOrder: depthai.ColorCameraProperties.ColorOrder)
Set color order of preview output images. RGB or BGR
method
setFp16(self, fp16: bool)
Set fp16 (0..255) data type of preview output frames
method
setFps(self, fps: float)
Set rate at which camera should produce frames Parameter ``fps``: Rate in frames per second
method
method
setImageOrientation(self, imageOrientation: depthai.CameraImageOrientation)
Set camera image orientation
method
setInterleaved(self, interleaved: bool)
Set planar or interleaved data of preview output frames
method
setIsp3aFps(self, isp3aFps: int)
Isp 3A rate (auto focus, auto exposure, auto white balance, camera controls etc.). Default (0) matches the camera FPS, meaning that 3A is running on each frame. Reducing the rate of 3A reduces the CPU usage on CSS, but also increases the convergence rate of 3A. Note that camera controls will be processed at this rate. E.g. if camera is running at 30 fps, and camera control is sent at every frame, but 3A fps is set to 15, the camera control messages will be processed at 15 fps rate, which will lead to queueing.
method
setIspNumFramesPool(self, arg0: int)
Set number of frames in isp pool
method
method
setNumFramesPool(self, raw: int, isp: int, preview: int, video: int, still: int)
Set number of frames in all pools
method
setPreviewKeepAspectRatio(self, keep: bool)
Specifies whether preview output should preserve aspect ratio, after downscaling from video size or not. Parameter ``keep``: If true, a larger crop region will be considered to still be able to create the final image in the specified aspect ratio. Otherwise video size is resized to fit preview size
method
setPreviewNumFramesPool(self, arg0: int)
Set number of frames in preview pool
method
method
setRawNumFramesPool(self, arg0: int)
Set number of frames in raw pool
method
setRawOutputPacked(self, packed: bool)
Configures whether the camera `raw` frames are saved as MIPI-packed to memory. The packed format is more efficient, consuming less memory on device, and less data to send to host: RAW10: 4 pixels saved on 5 bytes, RAW12: 2 pixels saved on 3 bytes. When packing is disabled (`false`), data is saved lsb-aligned, e.g. a RAW10 pixel will be stored as uint16, on bits 9..0: 0b0000'00pp'pppp'pppp. Default is auto: enabled for standard color/monochrome cameras where ISP can work with both packed/unpacked, but disabled for other cameras like ToF.
method
setResolution(self, resolution: depthai.ColorCameraProperties.SensorResolution)
Set sensor resolution
method
setSensorCrop(self, x: float, y: float)
Specifies the cropping that happens when converting ISP to video output. By default, video will be center cropped from the ISP output. Note that this doesn't actually do on-sensor cropping (and MIPI-stream only that region), but it does postprocessing on the ISP (on RVC). Parameter ``x``: Top left X coordinate Parameter ``y``: Top left Y coordinate
method
setStillNumFramesPool(self, arg0: int)
Set number of frames in preview pool
method
method
setVideoNumFramesPool(self, arg0: int)
Set number of frames in preview pool
method
method
setWaitForConfigInput(self, wait: bool)
Specify to wait until inputConfig receives a configuration message, before sending out a frame. Parameter ``wait``: True to wait for inputConfig message, false otherwise
property
frameEvent
Outputs metadata-only ImgFrame message as an early indicator of an incoming frame. It's sent on the MIPI SoF (start-of-frame) event, just after the exposure of the current frame has finished and before the exposure for next frame starts. Could be used to synchronize various processes with camera capture. Fields populated: camera id, sequence number, timestamp
property
initialControl
Initial control options to apply to sensor
property
inputConfig
Input for ImageManipConfig message, which can modify crop parameters in runtime Default queue is non-blocking with size 8
property
inputControl
Input for CameraControl message, which can modify camera parameters in runtime Default queue is blocking with size 8
property
isp
Outputs ImgFrame message that carries YUV420 planar (I420/IYUV) frame data. Generated by the ISP engine, and the source for the 'video', 'preview' and 'still' outputs
property
preview
Outputs ImgFrame message that carries BGR/RGB planar/interleaved encoded frame data. Suitable for use with NeuralNetwork node
property
raw
Outputs ImgFrame message that carries RAW10-packed (MIPI CSI-2 format) frame data. Captured directly from the camera sensor, and the source for the 'isp' output.
property
still
Outputs ImgFrame message that carries NV12 encoded (YUV420, UV plane interleaved) frame data. The message is sent only when a CameraControl message arrives to inputControl with captureStill command set.
property
video
Outputs ImgFrame message that carries NV12 encoded (YUV420, UV plane interleaved) frame data. Suitable for use with VideoEncoder node
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.