RGB scene¶
This example shows how to select ColorCamera scene and effect.
s will switch between available scenes
e will switch between available effects (currently not available)
Demo¶
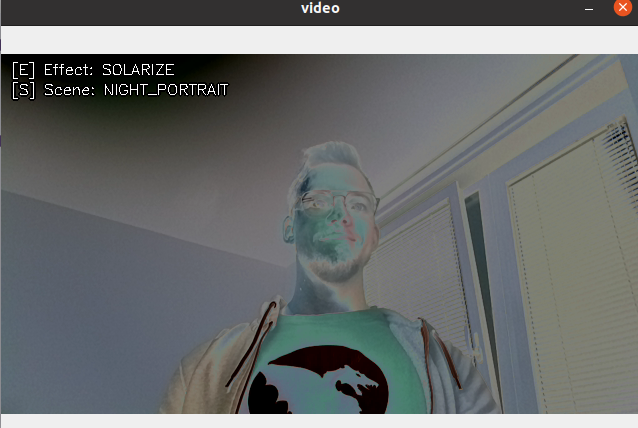
Setup¶
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the script
git clone https://github.com/luxonis/depthai-python.git
cd depthai-python/examples
python3 install_requirements.py
For additional information, please follow installation guide
Source code¶
Also available on GitHub
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | import cv2 import depthai as dai from itertools import cycle scenes = cycle([item for name, item in vars(dai.RawCameraControl.SceneMode).items() if name != "UNSUPPORTED" and name.isupper()]) effects = cycle([item for name, item in vars(dai.RawCameraControl.EffectMode).items() if name.isupper()]) curr_scene = "OFF" curr_effect = "OFF" # Create pipeline pipeline = dai.Pipeline() camRgb = pipeline.create(dai.node.ColorCamera) camRgb.setIspScale(1,3) xoutRgb = pipeline.create(dai.node.XLinkOut) xoutRgb.setStreamName("video") camRgb.video.link(xoutRgb.input) camControlIn = pipeline.create(dai.node.XLinkIn) camControlIn.setStreamName("camControl") camControlIn.out.link(camRgb.inputControl) # Connect to device and start pipeline with dai.Device(pipeline) as device: videoQ = device.getOutputQueue(name="video", maxSize=4, blocking=False) ctrlQ = device.getInputQueue(name="camControl") def putText(frame, text, coords): cv2.putText(frame, text, coords, cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 0), 4) cv2.putText(frame, text, coords, cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255, 255, 255), 1) while True: videoIn = videoQ.tryGet() if videoIn is not None: frame = videoIn.getCvFrame() putText(frame, f"[E] Effect: {curr_effect}", (10, 20)) putText(frame, f"[S] Scene: {curr_scene}", (10, 40)) cv2.imshow("video", frame) key = cv2.waitKey(1) if key == ord('e') or key == ord('E'): effect = next(effects) print("Switching colorCamera effect:", str(effect)) curr_effect = str(effect).lstrip("EffectMode.") cfg = dai.CameraControl() cfg.setEffectMode(effect) ctrlQ.send(cfg) # Scene currently doesn't work elif key == ord('s') or key == ord('S'): scene = next(scenes) print("Currently doesn't work! Switching colorCamera Scene:", str(scene)) curr_scene = str(scene).lstrip("SceneMode.") cfg = dai.CameraControl() cfg.setSceneMode(scene) ctrlQ.send(cfg) elif key == ord('q'): break |