Script camera control
This example shows how to use Script node. It controls the ColorCamera to capture a still image every second.Demo
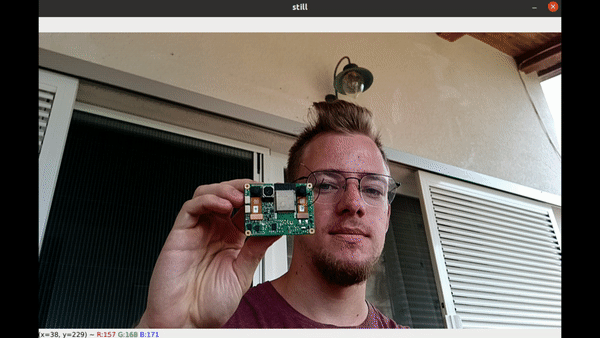
Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2import cv2
3import depthai as dai
4
5# Start defining a pipeline
6pipeline = dai.Pipeline()
7
8# Define a source - color camera
9cam = pipeline.create(dai.node.ColorCamera)
10
11# Script node
12script = pipeline.create(dai.node.Script)
13script.setScript("""
14 import time
15 ctrl = CameraControl()
16 ctrl.setCaptureStill(True)
17 while True:
18 time.sleep(1)
19 node.io['out'].send(ctrl)
20""")
21
22# XLinkOut
23xout = pipeline.create(dai.node.XLinkOut)
24xout.setStreamName('still')
25
26# Connections
27script.outputs['out'].link(cam.inputControl)
28cam.still.link(xout.input)
29
30# Connect to device with pipeline
31with dai.Device(pipeline) as device:
32 while True:
33 img = device.getOutputQueue("still").get()
34 cv2.imshow('still', img.getCvFrame())
35 if cv2.waitKey(1) == ord('q'):
36 break
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.