Disparity encoding
This example encodes disparity output of the StereoDepth. Note that you shouldn't enable subpixel mode, as UINT16 isn't supported by the VideoEncoder.Pressing Ctrl+C will stop the recording and then convert it using ffmpeg into an mp4 to make it playable. Note that ffmpeg will need to be installed and runnable for the conversion to mp4 to succeed.Be careful, this example saves encoded video to your host storage. So if you leave it running, you could fill up your storage on your host.Similar samples:
Demo
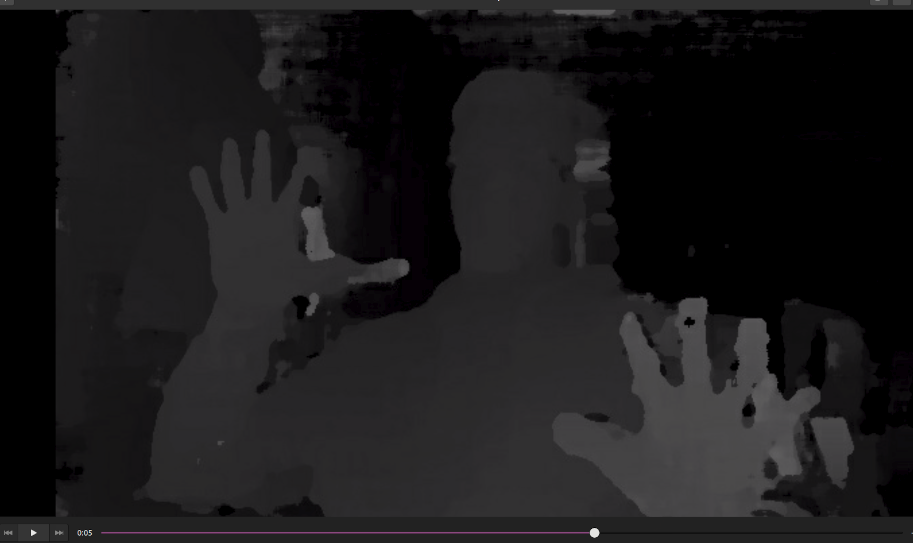
Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
PythonGitHub
1#!/usr/bin/env python3
2
3import depthai as dai
4
5# NOTE Because we are encoding disparity values, output video will be a gray, and won't have many pixel levels - either 0..95 or 0..190
6
7# Create pipeline
8pipeline = dai.Pipeline()
9
10# Create left/right mono cameras for Stereo depth
11monoLeft = pipeline.create(dai.node.MonoCamera)
12monoLeft.setResolution(dai.MonoCameraProperties.SensorResolution.THE_400_P)
13monoLeft.setCamera("left")
14
15monoRight = pipeline.create(dai.node.MonoCamera)
16monoRight.setResolution(dai.MonoCameraProperties.SensorResolution.THE_400_P)
17monoRight.setCamera("right")
18
19# Create a node that will produce the depth map
20depth = pipeline.create(dai.node.StereoDepth)
21depth.setDefaultProfilePreset(dai.node.StereoDepth.PresetMode.HIGH_DENSITY)
22depth.initialConfig.setMedianFilter(dai.MedianFilter.KERNEL_7x7)
23depth.setLeftRightCheck(False)
24depth.setExtendedDisparity(False)
25# Subpixel disparity is of UINT16 format, which is unsupported by VideoEncoder
26depth.setSubpixel(False)
27monoLeft.out.link(depth.left)
28monoRight.out.link(depth.right)
29
30videoEnc = pipeline.create(dai.node.VideoEncoder)
31# Depth resolution/FPS will be the same as mono resolution/FPS
32videoEnc.setDefaultProfilePreset(monoLeft.getFps(), dai.VideoEncoderProperties.Profile.MJPEG)
33depth.disparity.link(videoEnc.input)
34
35xout = pipeline.create(dai.node.XLinkOut)
36xout.setStreamName("enc")
37videoEnc.bitstream.link(xout.input)
38
39# Connect to device and start pipeline
40with dai.Device(pipeline) as device:
41
42 # Output queue will be used to get the encoded data from the output defined above
43 q = device.getOutputQueue(name="enc")
44
45 # The .h265 file is a raw stream file (not playable yet)
46 with open('disparity.mjpeg', 'wb') as videoFile:
47 print("Press Ctrl+C to stop encoding...")
48 try:
49 while True:
50 videoFile.write(q.get().getData())
51 except KeyboardInterrupt:
52 # Keyboard interrupt (Ctrl + C) detected
53 pass
54
55 print("To view the encoded data, convert the stream file (.mjpeg) into a video file (.mp4) using a command below:")
56 print("ffmpeg -framerate 30 -i disparity.mjpeg -c copy video.mp4")
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.