Casting NN Blur
This example demonstrates how to apply a blur effect using a neural network and the Cast node. The output of the cast node can be used in second stage neural networks or for further processing.Demo
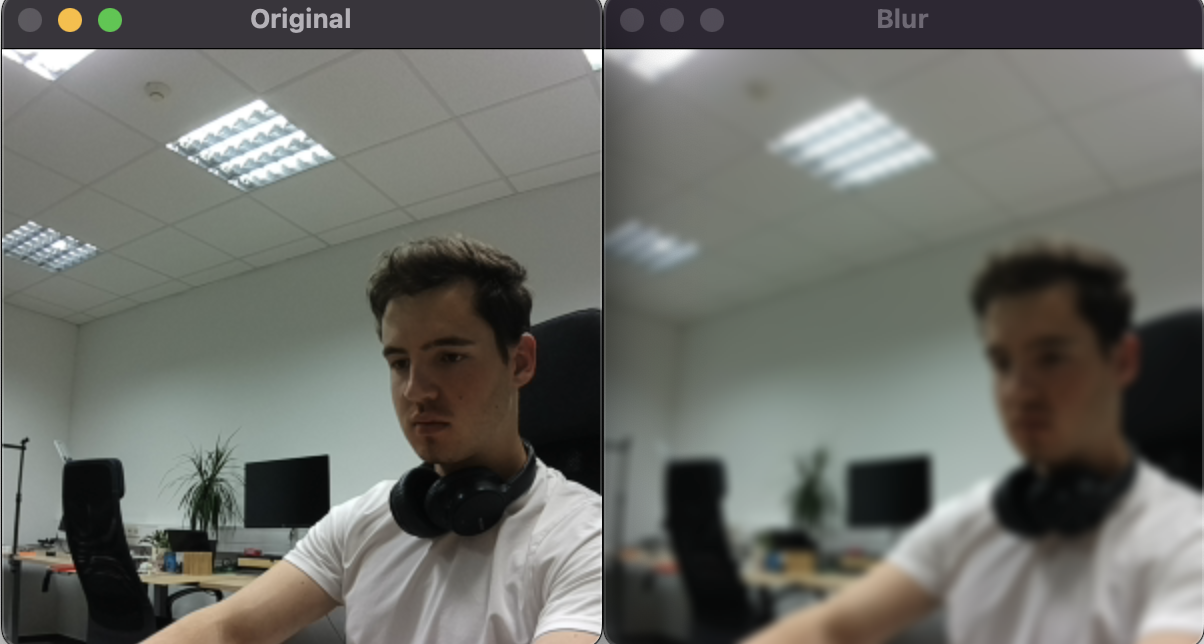
Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2
3import depthai as dai
4import cv2
5from pathlib import Path
6
7SHAPE = 300
8
9p = dai.Pipeline()
10
11camRgb = p.create(dai.node.ColorCamera)
12nn = p.create(dai.node.NeuralNetwork)
13rgbOut = p.create(dai.node.XLinkOut)
14cast = p.create(dai.node.Cast)
15castXout = p.create(dai.node.XLinkOut)
16
17camRgb.setPreviewSize(SHAPE, SHAPE)
18camRgb.setInterleaved(False)
19
20nnBlobPath = (Path(__file__).parent / Path('../models/blur_simplified_openvino_2021.4_6shave.blob')).resolve().absolute()
21
22nn.setBlobPath(nnBlobPath)
23
24rgbOut.setStreamName("rgb")
25
26castXout.setStreamName("cast")
27
28cast.setOutputFrameType(dai.RawImgFrame.Type.BGR888p)
29
30# Linking
31camRgb.preview.link(nn.input)
32camRgb.preview.link(rgbOut.input)
33nn.out.link(cast.input)
34cast.output.link(castXout.input)
35
36with dai.Device(p) as device:
37 qCam = device.getOutputQueue(name="rgb", maxSize=4, blocking=False)
38 qCast = device.getOutputQueue(name="cast", maxSize=4, blocking=False)
39
40
41 while True:
42 inCast = qCast.get()
43 assert isinstance(inCast, dai.ImgFrame)
44 inRgb = qCam.get()
45 assert isinstance(inRgb, dai.ImgFrame)
46 cv2.imshow("Blur", inCast.getCvFrame())
47 cv2.imshow("Original", inRgb.getCvFrame())
48
49
50 if cv2.waitKey(1) == ord('q'):
51 break
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.