ImageManip Rotate
This example showcases how to rotate color and mono frames with the help of ImageManip node. In the example, we are rotating by 90°.Due to HW warp constraint, input image (to be rotated) has to have width value of multiples of 16.
Demos
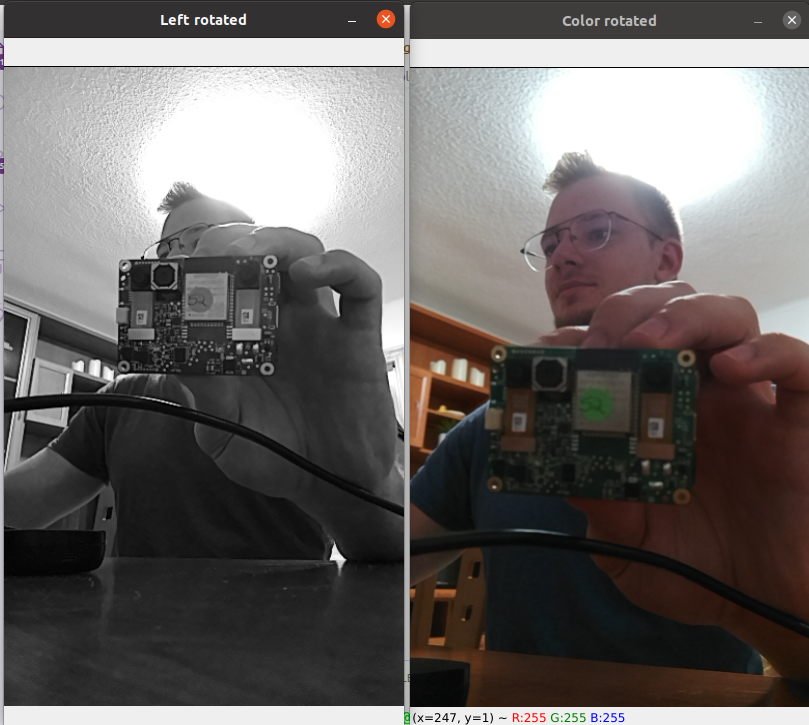
Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2
3import cv2
4import depthai as dai
5
6# Create pipeline
7pipeline = dai.Pipeline()
8
9# Rotate color frames
10camRgb = pipeline.create(dai.node.ColorCamera)
11camRgb.setPreviewSize(640, 400)
12camRgb.setResolution(dai.ColorCameraProperties.SensorResolution.THE_1080_P)
13camRgb.setInterleaved(False)
14
15manipRgb = pipeline.create(dai.node.ImageManip)
16rgbRr = dai.RotatedRect()
17rgbRr.center.x, rgbRr.center.y = camRgb.getPreviewWidth() // 2, camRgb.getPreviewHeight() // 2
18rgbRr.size.width, rgbRr.size.height = camRgb.getPreviewHeight(), camRgb.getPreviewWidth()
19rgbRr.angle = 90
20manipRgb.initialConfig.setCropRotatedRect(rgbRr, False)
21camRgb.preview.link(manipRgb.inputImage)
22
23manipRgbOut = pipeline.create(dai.node.XLinkOut)
24manipRgbOut.setStreamName("manip_rgb")
25manipRgb.out.link(manipRgbOut.input)
26
27# Rotate mono frames
28monoLeft = pipeline.create(dai.node.MonoCamera)
29monoLeft.setResolution(dai.MonoCameraProperties.SensorResolution.THE_400_P)
30monoLeft.setCamera("left")
31
32manipLeft = pipeline.create(dai.node.ImageManip)
33rr = dai.RotatedRect()
34rr.center.x, rr.center.y = monoLeft.getResolutionWidth() // 2, monoLeft.getResolutionHeight() // 2
35rr.size.width, rr.size.height = monoLeft.getResolutionHeight(), monoLeft.getResolutionWidth()
36rr.angle = 90
37manipLeft.initialConfig.setCropRotatedRect(rr, False)
38monoLeft.out.link(manipLeft.inputImage)
39
40manipLeftOut = pipeline.create(dai.node.XLinkOut)
41manipLeftOut.setStreamName("manip_left")
42manipLeft.out.link(manipLeftOut.input)
43
44with dai.Device(pipeline) as device:
45 qLeft = device.getOutputQueue(name="manip_left", maxSize=8, blocking=False)
46 qRgb = device.getOutputQueue(name="manip_rgb", maxSize=8, blocking=False)
47
48 while True:
49 inLeft = qLeft.tryGet()
50 if inLeft is not None:
51 cv2.imshow('Left rotated', inLeft.getCvFrame())
52
53 inRgb = qRgb.tryGet()
54 if inRgb is not None:
55 cv2.imshow('Color rotated', inRgb.getCvFrame())
56
57 if cv2.waitKey(1) == ord('q'):
58 break
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.