Multiple devices
For many applications it's useful to have multiple OAK cameras running at the same time, as more cameras can perceive more (of the world around them). Examples here would include:- Box measurement app, multiple cameras from multiple different perspectives could provide better dimension estimation
- People counter/tracker app, multiple cameras could count/track people across a large area (eg. shopping mall)
- Attaching multiple cameras on front/back/left/right side of your robot for full 360° vision, so your robot can perceive the whole area around it regardless of how it's positioned.
Demo
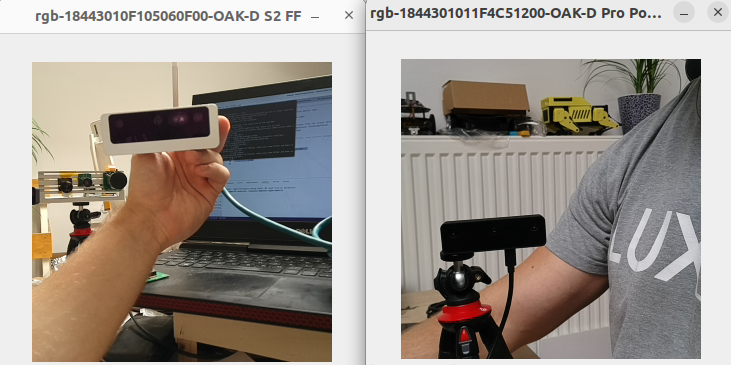
Command Line
1Connected to 18443010F105060F00
2 >>> MXID: 18443010F105060F00
3 >>> Num of cameras: 3
4 >>> USB speed: UsbSpeed.SUPER
5 >>> Board name: DM9098
6 >>> Product name: OAK-D S2 FF
7 Connected to 1844301011F4C51200
8 >>> MXID: 1844301011F4C51200
9 >>> Num of cameras: 3
10 >>> USB speed: UsbSpeed.UNKNOWN
11 >>> Board name: NG9097
12 >>> Product name: OAK-D Pro PoE AF
Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2
3import cv2
4import depthai as dai
5import contextlib
6
7def createPipeline():
8 # Start defining a pipeline
9 pipeline = dai.Pipeline()
10 # Define a source - color camera
11 camRgb = pipeline.create(dai.node.ColorCamera)
12
13 camRgb.setPreviewSize(300, 300)
14 camRgb.setBoardSocket(dai.CameraBoardSocket.CAM_A)
15 camRgb.setResolution(dai.ColorCameraProperties.SensorResolution.THE_1080_P)
16 camRgb.setInterleaved(False)
17
18 # Create output
19 xoutRgb = pipeline.create(dai.node.XLinkOut)
20 xoutRgb.setStreamName("rgb")
21 camRgb.preview.link(xoutRgb.input)
22
23 return pipeline
24
25
26with contextlib.ExitStack() as stack:
27 deviceInfos = dai.Device.getAllAvailableDevices()
28 usbSpeed = dai.UsbSpeed.SUPER
29 openVinoVersion = dai.OpenVINO.Version.VERSION_2021_4
30
31 qRgbMap = []
32 devices = []
33
34 for deviceInfo in deviceInfos:
35 deviceInfo: dai.DeviceInfo
36 device: dai.Device = stack.enter_context(dai.Device(openVinoVersion, deviceInfo, usbSpeed))
37 devices.append(device)
38 print("===Connected to ", deviceInfo.getMxId())
39 mxId = device.getMxId()
40 cameras = device.getConnectedCameras()
41 usbSpeed = device.getUsbSpeed()
42 eepromData = device.readCalibration2().getEepromData()
43 print(" >>> MXID:", mxId)
44 print(" >>> Num of cameras:", len(cameras))
45 print(" >>> USB speed:", usbSpeed)
46 if eepromData.boardName != "":
47 print(" >>> Board name:", eepromData.boardName)
48 if eepromData.productName != "":
49 print(" >>> Product name:", eepromData.productName)
50
51 pipeline = createPipeline()
52 device.startPipeline(pipeline)
53
54 # Output queue will be used to get the rgb frames from the output defined above
55 q_rgb = device.getOutputQueue(name="rgb", maxSize=4, blocking=False)
56 stream_name = "rgb-" + mxId + "-" + eepromData.productName
57 qRgbMap.append((q_rgb, stream_name))
58
59 while True:
60 for q_rgb, stream_name in qRgbMap:
61 if q_rgb.has():
62 cv2.imshow(stream_name, q_rgb.get().getCvFrame())
63
64 if cv2.waitKey(1) == ord('q'):
65 break
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.