Script forward frames
This example shows how to use Script node to forward (demultiplex) frames to two different outputs - in this case directly to two XLinkOut nodes. Script also changes exposure ratio for each frame, which results in two streams, one lighter and one darker.Demo
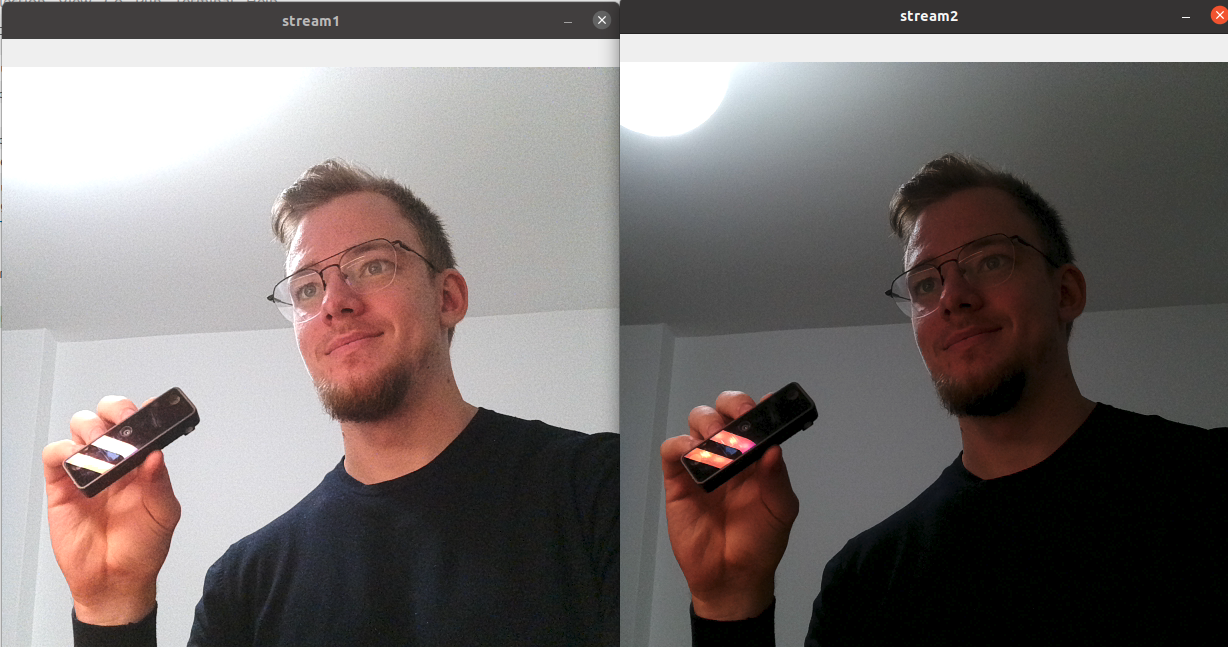
Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2import cv2
3import depthai as dai
4
5# Start defining a pipeline
6pipeline = dai.Pipeline()
7
8cam = pipeline.create(dai.node.ColorCamera)
9# Not needed, you can display 1080P frames as well
10cam.setIspScale(1,2)
11
12# Script node
13script = pipeline.create(dai.node.Script)
14script.setScript("""
15 ctrl = CameraControl()
16 ctrl.setCaptureStill(True)
17 # Initially send still event
18 node.io['ctrl'].send(ctrl)
19
20 normal = True
21 while True:
22 frame = node.io['frames'].get()
23 if normal:
24 ctrl.setAutoExposureCompensation(3)
25 node.io['stream1'].send(frame)
26 normal = False
27 else:
28 ctrl.setAutoExposureCompensation(-3)
29 node.io['stream2'].send(frame)
30 normal = True
31 node.io['ctrl'].send(ctrl)
32""")
33cam.still.link(script.inputs['frames'])
34
35# XLinkOut
36xout1 = pipeline.create(dai.node.XLinkOut)
37xout1.setStreamName('stream1')
38script.outputs['stream1'].link(xout1.input)
39
40xout2 = pipeline.create(dai.node.XLinkOut)
41xout2.setStreamName('stream2')
42script.outputs['stream2'].link(xout2.input)
43
44script.outputs['ctrl'].link(cam.inputControl)
45
46# Connect to device with pipeline
47with dai.Device(pipeline) as device:
48 qStream1 = device.getOutputQueue("stream1")
49 qStream2 = device.getOutputQueue("stream2")
50 while True:
51 cv2.imshow('stream1', qStream1.get().getCvFrame())
52 cv2.imshow('stream2', qStream2.get().getCvFrame())
53 if cv2.waitKey(1) == ord('q'):
54 break
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.