Script HTTP server
This example can only run on OAK POE devices. You need bootloader on/above version 0.0.15. You can flash bootloader by running
python3 examples/bootloader/flash_bootloader.py
.Demo
When you run the demo, it will print something similar toCommand Line
1Serving at 192.168.1.193:8080
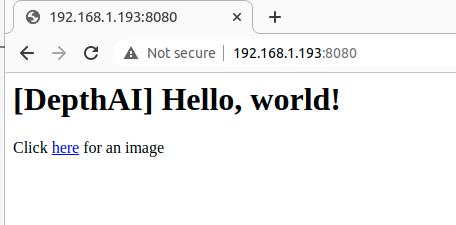
here
href, you will get a static image. For video stream, you should check out Script MJPEG server.Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2
3import depthai as dai
4import time
5
6# Start defining a pipeline
7pipeline = dai.Pipeline()
8
9# Define a source - color camera
10cam = pipeline.create(dai.node.ColorCamera)
11# VideoEncoder
12jpeg = pipeline.create(dai.node.VideoEncoder)
13jpeg.setDefaultProfilePreset(cam.getFps(), dai.VideoEncoderProperties.Profile.MJPEG)
14
15# Script node
16script = pipeline.create(dai.node.Script)
17script.setProcessor(dai.ProcessorType.LEON_CSS)
18script.setScript("""
19 from http.server import BaseHTTPRequestHandler
20 import socketserver
21 import socket
22 import fcntl
23 import struct
24
25 PORT = 8080
26 ctrl = CameraControl()
27 ctrl.setCaptureStill(True)
28
29 def get_ip_address(ifname):
30 s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
31 return socket.inet_ntoa(fcntl.ioctl(
32 s.fileno(),
33 -1071617759, # SIOCGIFADDR
34 struct.pack('256s', ifname[:15].encode())
35 )[20:24])
36
37 class HTTPHandler(BaseHTTPRequestHandler):
38 def do_GET(self):
39 if self.path == '/':
40 self.send_response(200)
41 self.end_headers()
42 self.wfile.write(b'<h1>[DepthAI] Hello, world!</h1><p>Click <a href="img">here</a> for an image</p>')
43 elif self.path == '/img':
44 node.io['out'].send(ctrl)
45 jpegImage = node.io['jpeg'].get()
46 self.send_response(200)
47 self.send_header('Content-Type', 'image/jpeg')
48 self.send_header('Content-Length', str(len(jpegImage.getData())))
49 self.end_headers()
50 self.wfile.write(jpegImage.getData())
51 else:
52 self.send_response(404)
53 self.end_headers()
54 self.wfile.write(b'Url not found...')
55
56 with socketserver.TCPServer(("", PORT), HTTPHandler) as httpd:
57 node.warn(f"Serving at {get_ip_address('re0')}:{PORT}")
58 httpd.serve_forever()
59""")
60
61# Connections
62cam.still.link(jpeg.input)
63script.outputs['out'].link(cam.inputControl)
64jpeg.bitstream.link(script.inputs['jpeg'])
65
66# Connect to device with pipeline
67with dai.Device(pipeline) as device:
68 while not device.isClosed():
69 time.sleep(1)
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.