Script MJPEG server
This example can only run on OAK POE devices. You need bootloader on/above version 0.0.15. You can flash bootloader by running
python3 examples/bootloader/flash_bootloader.py
.Demo
When you run the demo, it will print something similar toCommand Line
1Serving at 192.168.1.193:8080
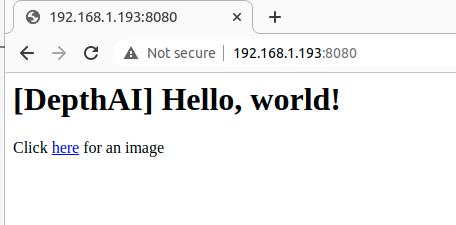
here
href, you will get the MJPEG video stream. For static image, you can check out Script HTTP server.Setup
Please run the install script to download all required dependencies. Please note that this script must be ran from git context, so you have to download the depthai-python repository first and then run the scriptCommand Line
1git clone https://github.com/luxonis/depthai-python.git
2cd depthai-python/examples
3python3 install_requirements.py
Source code
Python
C++
Python
PythonGitHub
1#!/usr/bin/env python3
2
3import depthai as dai
4import time
5
6# Start defining a pipeline
7pipeline = dai.Pipeline()
8
9# Define a source - color camera
10cam = pipeline.create(dai.node.ColorCamera)
11# VideoEncoder
12jpeg = pipeline.create(dai.node.VideoEncoder)
13jpeg.setDefaultProfilePreset(cam.getFps(), dai.VideoEncoderProperties.Profile.MJPEG)
14
15# Script node
16script = pipeline.create(dai.node.Script)
17script.setProcessor(dai.ProcessorType.LEON_CSS)
18script.setScript("""
19 import time
20 import socket
21 import fcntl
22 import struct
23 from socketserver import ThreadingMixIn
24 from http.server import BaseHTTPRequestHandler, HTTPServer
25
26 PORT = 8080
27
28 def get_ip_address(ifname):
29 s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
30 return socket.inet_ntoa(fcntl.ioctl(
31 s.fileno(),
32 -1071617759, # SIOCGIFADDR
33 struct.pack('256s', ifname[:15].encode())
34 )[20:24])
35
36 class ThreadingSimpleServer(ThreadingMixIn, HTTPServer):
37 pass
38
39 class HTTPHandler(BaseHTTPRequestHandler):
40 def do_GET(self):
41 if self.path == '/':
42 self.send_response(200)
43 self.end_headers()
44 self.wfile.write(b'<h1>[DepthAI] Hello, world!</h1><p>Click <a href="img">here</a> for an image</p>')
45 elif self.path == '/img':
46 try:
47 self.send_response(200)
48 self.send_header('Content-type', 'multipart/x-mixed-replace; boundary=--jpgboundary')
49 self.end_headers()
50 fpsCounter = 0
51 timeCounter = time.time()
52 while True:
53 jpegImage = node.io['jpeg'].get()
54 self.wfile.write("--jpgboundary".encode())
55 self.wfile.write(bytes([13, 10]))
56 self.send_header('Content-type', 'image/jpeg')
57 self.send_header('Content-length', str(len(jpegImage.getData())))
58 self.end_headers()
59 self.wfile.write(jpegImage.getData())
60 self.end_headers()
61
62 fpsCounter = fpsCounter + 1
63 if time.time() - timeCounter > 1:
64 node.warn(f'FPS: {fpsCounter}')
65 fpsCounter = 0
66 timeCounter = time.time()
67 except Exception as ex:
68 node.warn(str(ex))
69
70 with ThreadingSimpleServer(("", PORT), HTTPHandler) as httpd:
71 node.warn(f"Serving at {get_ip_address('re0')}:{PORT}")
72 httpd.serve_forever()
73""")
74
75# Connections
76cam.video.link(jpeg.input)
77jpeg.bitstream.link(script.inputs['jpeg'])
78
79# Connect to device with pipeline
80with dai.Device(pipeline) as device:
81 while not device.isClosed():
82 time.sleep(1)
Pipeline
Need assistance?
Head over to Discussion Forum for technical support or any other questions you might have.